Python Desktop Audio Record Loopback
2024-07-21
筆記如何使用 Python 錄製作業系統桌面環境音效與迴路輸出。
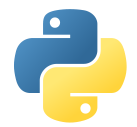
說明
參考 tez3998 於 GitHub 所分享的專案 loopback-capture-sample。
主要透過 soundcard
以及 soundfile
來進行錄製與寫入的作業,同時因為錄製是指定時間長度,自行增加一個函數來清除結尾的空白。
import soundcard as sc
import soundfile as sf
import uuid
import numpy as np
OUTPUT_FILE_NAME = f'{uuid.uuid4()}.wav' # file name.
SAMPLE_RATE = 48000 # [Hz]. sampling rate.
RECORD_SEC = 20 # [sec]. duration recording audio.
def read_and_cutoff(file_path, threshold=0.01):
# Read audio file
data, samplerate = sf.read(file_path)
# Calculate the absolute value of the audio
abs_data = np.abs(data)
# Traverse from the end to find the first point that exceeds the threshold
for i in range(len(abs_data) - 1, 0, -1):
if np.any(abs_data[i] > threshold):
break
# Cut the audio data to remove the silent tail
cutoff_data = data[:i+1]
# Write to a new file
sf.write(file_path, cutoff_data, samplerate)
def record_start():
with sc.get_microphone(id=str(sc.default_speaker().name), include_loopback=True).recorder(samplerate=SAMPLE_RATE) as mic:
# record audio with loopback from default speaker.
data = mic.record(numframes=SAMPLE_RATE*RECORD_SEC)
# change "data=data[:, 0]" to "data=data", if you would like to write audio as multiple-channels.
sf.write(file=OUTPUT_FILE_NAME, data=data[:, 0], samplerate=SAMPLE_RATE)
record_start()
read_and_cutoff(OUTPUT_FILE_NAME)