綜整日常 Python 開發慣用的 Snippets ,讓開發保持沉浸在心流不斷的快感 🐱👤
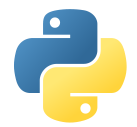
Python 101 🐍
Text file read & write
讀寫 txt file 的起手式。
def saveTxt(fileName = 'f.txt'):
with open(fileName, 'w', encoding = 'utf-8') as f:
f.writelines('text')
def loadTxt(fileName = 'f.txt'):
with open(fileName, encoding = 'utf-8') as f:
return f.readlines()
Print Number in Binary
print("{0:b}".format(7))
Import Modules 📦
Import BeauifulSoup to parse HTML / XML
載入 BeauifulSoup 處理爬下來的 HTML 資料起手式。
from bs4 import BeautifulSoup
soup = BeautifulSoup(content, 'lxml')
eautifulSoup(s.text.encode().decode('utf-8'), 'html.parser')
Import Colorama for CLI Colorful Output
載入 Colorama 用來控制 CLI 輸出的內容色彩。
from colorama import Style, Fore, Back
Import argparse for handle CLI args
載入 argparse 讓設計 CLI Tools 更為簡易。
import argparse
parser = argparse.ArgumentParser(description='')
parser.add_argument('-flag', action='store_true')
args = parser.parse_args()
Import hashlib, Hash strings with md5 5
import hashlib
hashlib.md5('content'.encode('utf-8')).hexdigest()
Import shutil, How to zip file
如何壓縮檔案為 zip
import shutil
shutil.make_archive(r'path\filename', 'zip', r'toZipPath\')
Datetime Magician 📅
Datetime Handle
如何將 Datetime Object 轉會為特別的字串形式。
datetime.datetime.now().strftime("%Y-%m-%d %H:%M:%S")
取得民國年 / 月 / 日
"".join([
v if int(v) < 2000 else str(int(v) - 1911)
for v in datetime.datetime.now().strftime("%Y-%m%d").split("-")
]
)
Advanced Data Structures 🧊
How to Sort Dictionary object
如何排序 Dict Object
sorted(_dcit.items(), key=lambda d: d[1], reverse = True)
Default Dictionary
from collections import defaultdict
dicWithDefaultList = defaultdict(list)
NamedTuple
from collections import namedtuple
EMPLOYEE = namedtuple('EMPLOYEE', ['name', 'pid'])
Python GroupBy
city_list = [
('TW', 'Taipei'), ('TW', 'Kaohsiung'),
('JP', 'Tokyo'),
('US', 'NewYork'),
('FR', 'Paris'), ('FR', 'Lille')
]
list(itertools.groupby(city_list, lambda ele : ele[0]))
#[('TW', <itertools._grouper at 0x121880af6d8>),
#('JP', <itertools._grouper at 0x121880af0f0>),
#('US', <itertools._grouper at 0x121880af5f8>),
#('FR', <itertools._grouper at 0x121880af400>)]
Automation 🤖
Automation Keyboard Control
import time, pyautogui, random
while True:
time.sleep(random.randint(3, 5))
pyautogui.hotkey('left')
time.sleep(random.randint(1, 3))
pyautogui.hotkey('win', 'prtscr')
Pending To Archive 🗝️
python encoding
set PYTHONIOENCODING=utf8