C# Python Bilingual - Data-Structures
2024-01-09
經常左手寫 C# 右手寫 Python,當你經常使用C#和Python這兩種編程語言時,你可能會發現它們在處理數據結構方面有著不同的特點和優勢。C# 具有強大 的LINQ Query,而 Python 以其靈活的資料結構而聞名。我將比較 Python 和 C# 在資料結構的相似之處,讓切換書寫的過程更為順暢 😀
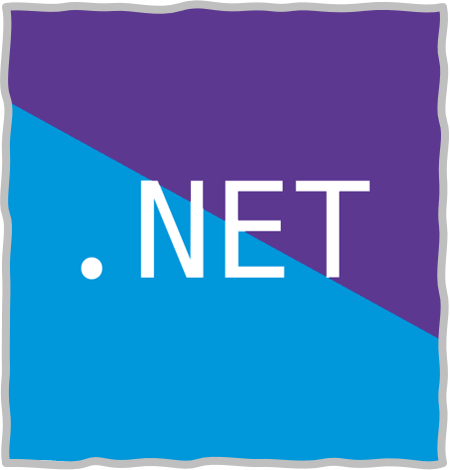
說明
C# | Python |
---|---|
List |
List |
HashSet |
Set |
Dictionary<TKey, TValue> | Dict |
ValueTuple<T1, T2, ...> | Tuple |
Struct | namedtuple |
# Using list
my_list = [1, 2, 3, 4, 5]
# Using set
my_set = set([1, 2, 2, 3, 4])
# Using dict
my_dict = {'apple': 1, 'banana': 2, 'cherry': 3}
# Using tuple
my_tuple = (1, 'apple', 3.14)
from collections import namedtuple
Point = namedtuple('Point', ['x', 'y'])
p1 = Point(1, 2)
p2 = Point(3, 4)
print(f'p1.x = {p1.x}, p1.y = {p1.y}')
using System;
using System.Collections.Generic;
// Using List<T>
List<int> myList = new List<int> { 1, 2, 3, 4, 5 };
// Using HashSet<T>
HashSet<int> mySet = new HashSet<int> { 1, 2, 2, 3, 4 };
// Using Dictionary<TKey, TValue>
Dictionary<string, int> myDict = new Dictionary<string, int>
{
{ "apple", 1 },
{ "banana", 2 },
{ "cherry", 3 }
};
// Using ValueTuple
ValueTuple<int, string, double> myTuple = (1, "apple", 3.14);
public struct Point
{
public int x;
public int y;
public Point(int x, int y)
{
this.x = x;
this.y = y;
}
}
Point p1 = new Point(1, 2);
Point p2 = new Point(3, 4);
Console.WriteLine($"p1.x = {p1.x}, p1.y = {p1.y}");