筆記如何處理 Blazor 父子元件之間參數傳遞與事件處理,讓演算法行為可以封裝在子元件當中達成重複使用的效益 😎
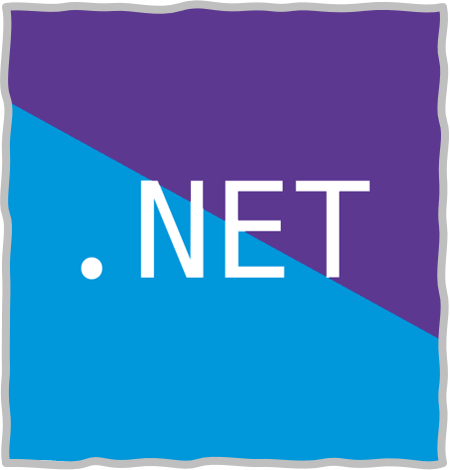
說明
ParentComponent.cs
@page "/page1"
<div>MyProperty @MyProperty</div>
<ClickButton CallbackMethod="UpdateProperty" CurrentValue="MyProperty" BtnDisplay="ChildComponent" />
@code {
private int MyProperty { get; set; }
private void RandomValue()
{
var rand = new Random();
MyProperty = rand.Next(1, 100);
}
private void UpdateProperty(int value)
{
MyProperty = value;
}
}
ChildComponent.cs
<div class="btn @BtnColor" @onclick="Callback">@BtnDisplay</div>
@code {
[Parameter]
public string? BtnDisplay { get; set; }
[Parameter]
public string? BtnColor { get; set; } = "btn-outline-info";
[Parameter]
public int CurrentValue { get; set; }
[Parameter]
public EventCallback<int> CallbackMethod { get; set; }
private int BtnAlgorithm()
{
return CurrentValue + 1;
}
private async Task Callback()
{
CurrentValue = BtnAlgorithm();
await CallbackMethod.InvokeAsync(CurrentValue);
}
}
如果要進一步有多個子元件,子元件本身有相同的 HTML Template 但差別的演算法,可以藉由 @inherits
的方式達成 😀
<!-- BaseComponent.razor -->
<h3>Base Component</h3>
<p>This is the base component.</p>
<!-- DerivedComponent.razor -->
@inherits BaseComponent
<h3>Derived Component</h3>
<p>This is the derived component.</p>
<!-- You can add additional content or customize behavior here. -->