Python 實用有趣又好玩的 Library
2023-12-28
筆記 Python 各式實用有趣又好玩的 Library,方便工作與流程的處理,豐富生活與科技結合的樂趣。
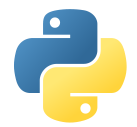
說明
使用 XlWings 處理 Excel
import xlwings as xw
# Step 1: Create a new Excel workbook
wb = xw.Book()
# Step 2: Access the default worksheet
sheet = wb.sheets['Sheet1']
# Step 3: Write headers to Excel
sheet.range('A1').value = 'Name'
sheet.range('B1').value = 'Age'
# Step 4: Add sample data
data = [
['John', 30],
['Alice', 25],
['Bob', 35],
]
sheet.range('A2').value = data
# Step 5: Read data from Excel
read_data = sheet.range('A1:B4').value
print("Data read from Excel:")
for row in read_data:
print(row)
# Step 6: Save and close the workbook
wb.save('example.xlsx') # Save the workbook as 'example.xlsx'
wb.close()
使用 Matplotlib 繪圖
import xlwings as xw
import matplotlib.pyplot as plt
# Step 1: Create a new Excel workbook
wb = xw.Book()
# Step 2: Access the default worksheet
sheet = wb.sheets['Sheet1']
# Step 3: Write headers and data to Excel
sheet.range('A1').value = 'Name'
sheet.range('B1').value = 'Age'
data = [
['John', 30],
['Alice', 25],
['Bob', 35],
]
sheet.range('A2').value = data
# Step 4: Read data from Excel
read_data = sheet.range('A1:B4').value
# Step 5: Create a Matplotlib plot
names = [row[0] for row in read_data[1:]]
ages = [row[1] for row in read_data[1:]]
plt.bar(names, ages)
plt.xlabel('Name')
plt.ylabel('Age')
plt.title('Age Distribution')
plt.show()
# Step 6: Save and close the workbook
wb.save('example.xlsx')
wb.close()
使用 Pyechart 繪圖
pip install pyecharts
from pyecharts import options as opts
from pyecharts.charts import Bar
# Step 1: Create data for the bar chart
x_data = ["Category A", "Category B", "Category C", "Category D", "Category E"]
y_data = [120, 200, 150, 80, 250]
# Step 2: Create a Bar chart object and configure its attributes
bar = (
Bar()
.add_xaxis(x_data)
.add_yaxis("Data", y_data)
.set_global_opts(title_opts=opts.TitleOpts(title="Bar Chart Example"))
)
# Step 3: Render the chart to an HTML file
bar.render("bar_chart.html")
使用 wxPython 設計 GUI App
import wx
# Step 1: Create a new wx.App object
app = wx.App(False)
# Step 2: Create a wx.Frame (main window) for the application
frame = wx.Frame(None, wx.ID_ANY, "Hello World App")
# Step 3: Add a wx.Panel to the frame
panel = wx.Panel(frame, wx.ID_ANY)
# Step 4: Add a wx.StaticText widget to the panel
text = wx.StaticText(panel, wx.ID_ANY, "Hello, wxPython!", pos=(10, 10))
# Step 5: Show the main window
frame.Show(True)
# Step 6: Start the main event loop
app.MainLoop()
⭐ Alternative Option 使用 pyQt5 設計 GUI App
使用 OpenCV 處理圖形
pip install opencv-python
import cv2
# Step 1: Load an image from a file
image = cv2.imread('image.jpg')
# Step 2: Display the loaded image
cv2.imshow('Original Image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
# Step 3: Convert the image to grayscale
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Step 4: Save the grayscale image to a file
cv2.imwrite('gray_image.jpg', gray_image)
使用 Tesseract 圖形辨識
from PIL import Image
import pytesseract
# Step 1: Open an image using Pillow
image = Image.open('image.png')
# Step 2: Perform text recognition using Tesseract
text = pytesseract.image_to_string(image)
# Step 3: Print the recognized text
print("Recognized Text:")
print(text)
使用 Wordcloud 製作文字雲
pip install wordcloud matplotlib
from wordcloud import WordCloud
import matplotlib.pyplot as plt
# Step 1: Create or load text data
text = "This is a simple example of creating a word cloud using Python. Word clouds are fun and informative."
# Step 2: Generate a word cloud
wordcloud = WordCloud(width=800, height=400).generate(text)
# Step 3: Display the word cloud using matplotlib
plt.figure(figsize=(10, 5))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis("off")
plt.show()