筆記如何使用 C# Dictionary 迭代呈現資料。
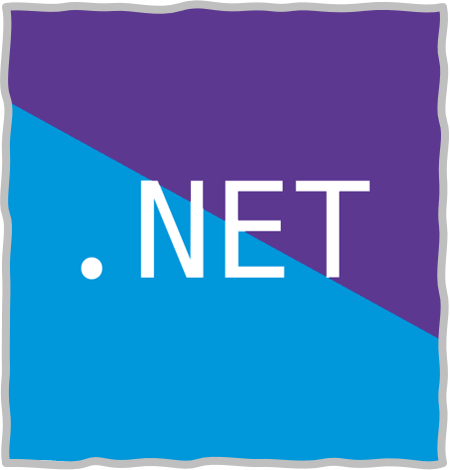
說明
Initializer
建構物件後,使用 Add
方法加入資料
var colorDict = new Dictionary<string, string>();
colorDict.Add("--bs-blue", "#0d6efd");
colorDict.Add("--bs-indigo", "#6610f2");
colorDict.Add("--bs-purple", "#6f42c1");
或者直接使用 collection initializer (Compiler 會自動呼叫對應的 Add
進行處理):
var colorDict = new Dictionary<string, string>()
{
{"--bs-blue", "#0d6efd"},
{"--bs-indigo", "#6610f2"},
{"--bs-purple", "#6f42c1"}
};
另一種 collection initializer 方式 (使用 public read / write indexer method):
var colorDict = new Dictionary<string, string>()
{
["--bs-blue"] = "#0d6efd",
["--bs-indigo"] = "#6610f2",
["--bs-purple"] = "#6f42c1"
};
TryGetValue
使用 TryGetValue 輸入 Key 值,搭配 out 如果 Key 存在將 Value 存入 out 的變數,如果不再則會回傳該型別的預設值 (int 為 0, string 為 null),如果想要客製化預設值,可以搭配 trinity operator。
string colorCodes = "";
colorDict.TryGetValue("--bs-blue2", out colorCodes) == true ?
colorCodes : "#fff";
Loop over dictionary
foreach (KeyValuePair<string, string> kvp in colorDict)
{
Console.WriteLine(kvp.Key, kvp.Value.ToUpper())
}
或者是更為簡便的方式:
foreach (var kvp in colorDict)
{
Console.WriteLine(kvp.Key, kvp.Value.ToUpper())
}
如果只需要 Dictionary 的 Keys,可以直接將 Key 以 ICollection
的方式取得:
foreach (var key in colorDict.Keys)
{
Console.WriteLine(key);
}
需要 Values 的話,也是相同的方式:
foreach (var value in colorDict.Values)
{
Console.WriteLine(value);
}