筆記由 GitHub 《30 seconds of C#》所學習到的語言使用技巧。
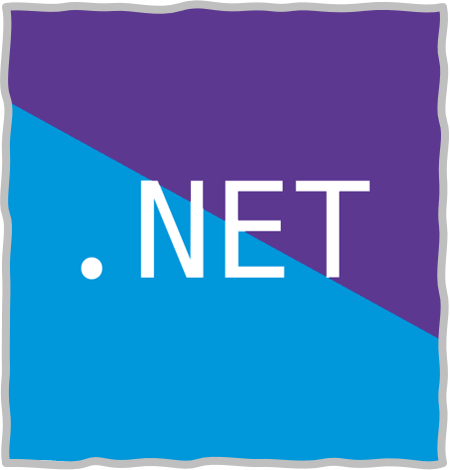
說明
Array.TrueForAll
藉由 Array.TrueForAll Static Method 可以判斷陣列中的物件是否符合 Predicate,達到 Python ALL
的同等功能。
var nums = new List<int>{ 3, 5, 7, 9, 11 };
bool isMatchPredicate = Array.TrueForAll(nums.ToArray(), x => x > 5);
Func<T, type>
藉由 Func<T, type>
可以將 lambda function 進行宣告,並且在傳入 Linq 當中。
List<Person> people = new List<Person>
{
new Person(){Height = 180, Weight = 75},
new Person(){Height = 160, Weight = 75},
new Person(){Height = 180, Weight = 55}
};
Func<Person, float> BMICalculateFunc = v => v.Weight / (v.Height * v.Height / 10000);
var peopleBMIList = people.Select(BMICalculateFunc).ToList();
進位制轉換, Convert
int number = 112;
Console.WriteLine(Convert.ToString(number, 2));
//1110000
byte[] bytes = { 64, 128, 255};
Console.WriteLine(BitConverter.ToString(bytes));
//40-80-FF
ToCharArray
因為 string 為 immutable ,所以如果要操作個別字元必須轉為 CharArray。
Chunk, 使用 Linq 分割 Collection
var data = new List<int> { 1, 2, 3, 4, 5 };
var chunks =
data
.Select((x, i) => new { Index = i, Value = x })
.GroupBy(x => x.Index / 2)
.Select(x => x.Select(v => v.Value).ToList())
.ToList();
foreach (var chunk in chunks)
{
Console.WriteLine(string.Join(",", chunk));
}
IEnumerable Extension Method : Except, Intersect
var data1 = new List<int> { 1, 3, 5 };
var data2 = new List<int> { 2, 4, 5 };
IEnumerable<int> except = data1.Except(data2);
Console.WriteLine("Except:");
foreach (var item in except)
{
Console.WriteLine(item);
}
// 1, 3
IEnumerable<int> intersect = data1.Intersect(data2);
Console.WriteLine("Intersect:");
foreach (var item in intersect)
{
Console.WriteLine(item);
}
// 5
Duplicate, 使用 Linq 找出重複的項目
int[] items = {1, 2, 1, 3, 2, 4};
var data = items
.GroupBy(c => c)
.Where(g => g.Count() > 1)
.Select(i => i.Key);
Array.FindAll
利用 Array.FindAll 可以簡易的過濾文字內容