超簡單學習 Vue.js 系列 | Fetch With Json Server
2021-12-28
Vue.js 簡單的示範專案,使用 Vue CLI 並搭配 Fetch API 向 Json Server 讀、寫、刪以及更新資料。
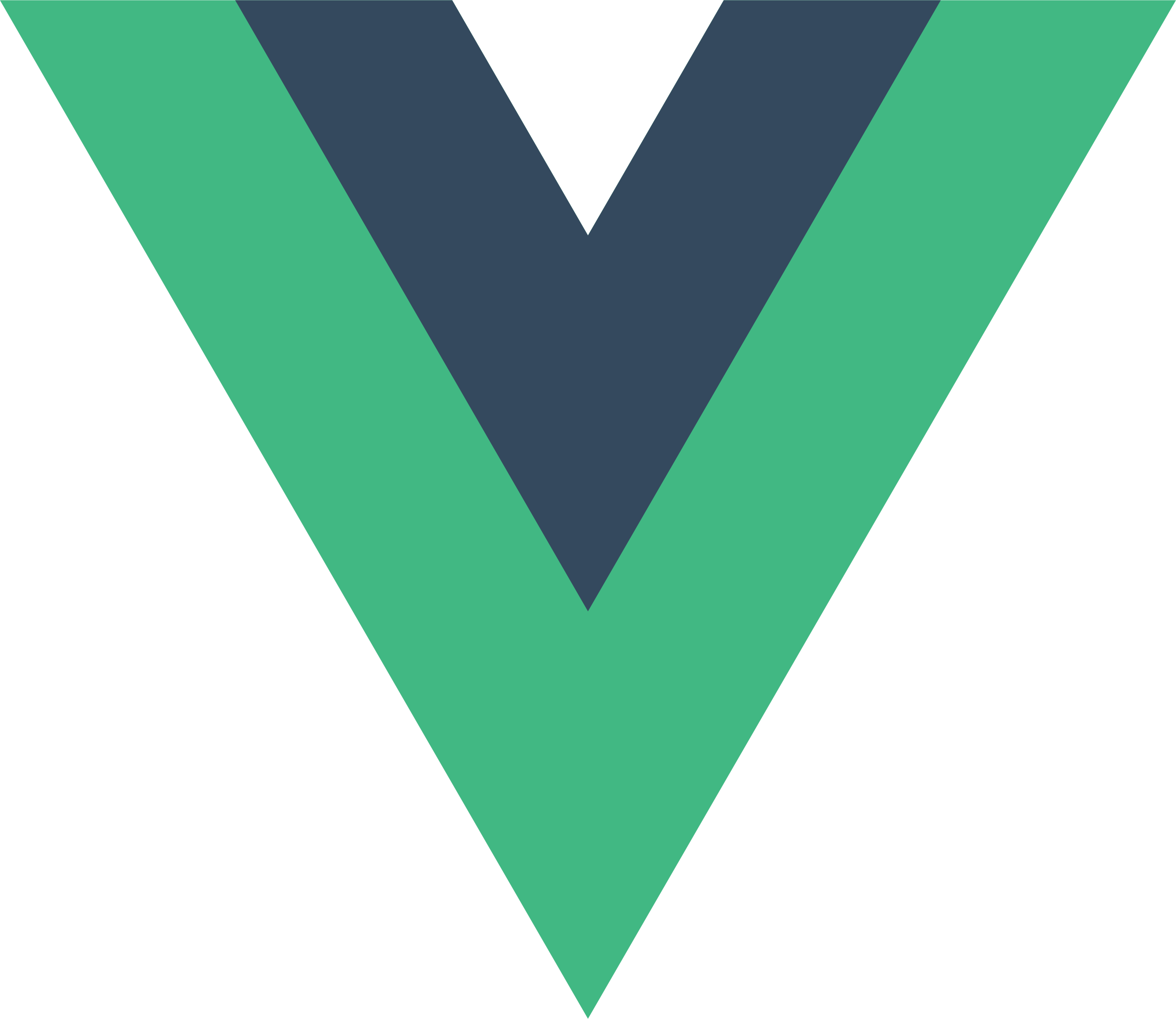
Fetch API
首先在專案中安裝 Json Servers 以及 bootstrap
npm install json-server
npm install bootstrap
Template
設計 Vue 的 template
<template>
<h1>Play Fetch With Json Server</h1>
<div style="min-height: 150px;" id="datablock">
<div v-for="post in posts" :key="post">
{{ post.id }} / {{ post.title }} / {{ post.author}}
</div>
</div>
<div class="btn" @click="fetchData">Fetch Data</div>
<div class="btn" @click="RemoveData(1)">Remove Data</div>
<div class="btn" @click="CreateData">Create Data</div>
<div class="btn" @click="UpdateData">Update Data</div>
</template>
<style>
@import'~bootstrap/dist/css/bootstrap.css';
</style>
上述的 template 省略顯示下列的 class 以免排版過亂,用途僅為美觀修飾。
Elements | CSS Class |
---|---|
#datablock | bg-danger |
#datablock | w-75 |
#datablock | mx-auto |
#datablock | p-3 |
#datablock | text-white |
#datablock | rounded |
div.btn | btn-outline-success |
div.btn | mx-3 |
div.btn | my-2 |
Json Server
db.json
{
"posts": [
{
"id": 1,
"title": "Fist Article",
"author": "S"
},
{
"id": 2,
"title": "Second Lieutenant",
"author": "S"
}
]
}
cd \db
json-server --watch db.json --port 8888
Fetch API
export default {
name: 'App',
data(){
return {
posts: []
}
},
methods: {
...
}
Methods 中具體的 Function 則如下:
Get / Read
要注意搭配 then 先將結果取為 json 再使用一次 then 更新 data。
fetchData(){
fetch('http://localhost:8888/posts')
.then(res => res.json())
.then(data => (this.posts = data))
},
Delete / Remove
參數 id 用於指定要刪除的項目,本案例是以 lastOne 資料中的最後一筆為例。
RemoveData(id){
const lastOne = Math.max(...this.posts.map(i => i.id))
fetch('http://localhost:8888/posts/' + lastOne, {
method: 'DELETE'
})
.then(res => res.json())
.then(() => this.posts = this.posts.filter(p => p.id != lastOne))
},
Create / Insert
Create 要注意要給予 headers 同時 body 的內容必須經由 JSON.stringify。
CreateData(){
const lastOne = Math.max(...this.posts.map(i => i.id))
let post = {
title: 'Hello World ' + Math.round(Math.random() * 100),
author: 'S',
id: lastOne + 1
}
fetch('http://localhost:8888/posts', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify(post)
}).then(() => this.posts.push(post))
},
Update / Edit
使用 PATCH Method 可以達到局部更新的使用,謝謝你 Json Server 😀
UpdateData(){
const lastOne = Math.max(...this.posts.map(i => i.id))
fetch('http://localhost:8888/posts/' + lastOne, {
method: 'PATCH',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ author: 'Edited'})
}).then(() => this.fetchData())
}