C# Console 學習筆記
2020-04-27
C# is good to use and good to learn.
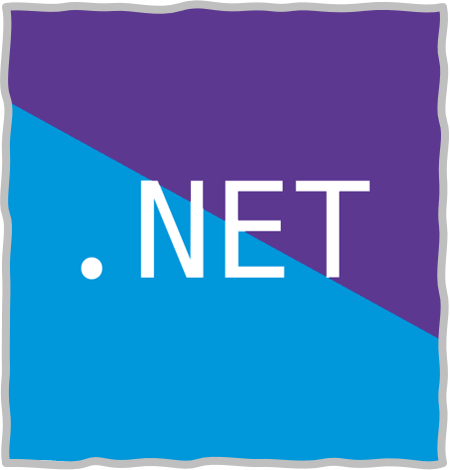
綜合
存取 App.config Key
步驟1:加入參考:System.Configuration
步驟2:設定 App.config
<configuration>
<appSettings>
<add key="configKey" value="configValue"/>
</appSettings>
</configuration>
步驟3:程式引用 config 值
using System.Configuration;
static void Main(string[] args)
{
Console.WriteLine(ConfigurationManager.AppSettings["configKey"]);
}
資料庫存取
⚡如果要 Insert String 包含中文等文字,必須要在 quote mark 之前加入N。
Insert Into tbName Values(N'櫻花', N'さくら');
🦄 否則必須使用 SqlCommand.Parameters
SqlCommand cmd = new SqlCommand();
cmd.CommandText = "insert into tbName (item) values (@flower)";
cmd.Parameters.Add("@flower", SqlDbType.NVarChar).Value = "さくら";
資料庫存取的範例
using System.Data.SqlClient;
static void Main(string[] args)
{
string Constr = "Persist Security Info=False; Integrated Security=true; \
Initial Catalog=...;Server=...;";
SqlConnection dataConnection = new SqlConnection { ConnectionString = Constr };
dataConnection.Open();
SqlCommand mySqlCmd = new SqlCommand("Insert into ...", dataConnection);
mySqlCmd.ExecuteNonQuery();
dataConnection.Close();
}
IO 資料讀入
string text = System.IO.File.ReadAllText(@"C:\filePath\file.txt",
System.Text.Encoding.Default);
Struct
struct屬於value type,因此在複製時是直接複製一份而非複製參考。而value type不可以被指為null,反之,reference type則可以。除非是nullable value type。
public struct Point { public int X, Y; }
static void Main()
{
Point p1 = new Point();
p1.X = 7;
Point p2 = p1; // Assignment causes copy
Console.WriteLine (p1.X); // 7
Console.WriteLine (p2.X); // 7
p1.X = 9; // Change p1.X
Console.WriteLine (p1.X); // 9
Console.WriteLine (p2.X); // 7
}
存取 Local JSON File
using System.IO;
using Newtonsoft.Json;
public static List<AuthMember> readJson()
{
var r = new List<AuthMember>{};
using (var sr = new StreamReader(
System.Web.HttpContext.Current.Server.MapPath("~/App_Data/member.json")))
{
r = JsonConvert.DeserializeObject<List<AuthMember>>(sr.ReadToEnd());
}
return r;
}
大數運算
using System.Numerics;
BigInteger bigIntFromInt64 = new BigInteger(12345678901234567890);
Console.WriteLine(bigIntFromInt64);
string positiveString = "123456789012345678901234567890";
BigInteger posBigInt = BigInteger.Parse(positiveString);
Console.WriteLine(posBigInt);
😋
物件導向
物件的建構
避免濫用 Constructor Overloadding ,如果只是不同 Fileds 的建構情境用 Object Initializer 替代,Object Initializer 是使用不含引數格式的建構子來進行,如果當此建構子不能被存取,使用 Object Initializer 就會發生錯誤。
public class Point{
public int X {get; set;}
public int Y {get; set;}
}
new Point{ X = 5, Y = 15};
MSDN - How to initialize objects by using an object initializer
建構子的繼承 / 呼叫父類別的建構子 Constructor Inheritance
public class Subclass : Baseclass
{
public Subclass (int x) : base (x) { }
}
is / as Operator
- is : 判斷變數是否指向特定型別(明確指定)
- as : 將型別轉型,若轉型失敗會指向null而非拋出錯誤
Virtual / Override / Sealed
class 方法有被 virtual 修飾的可以被繼承的 sub class override,如果沒有特別指定 virtual 與 override 則 sub class 會隱藏 pareant method。若方法被 sealed override,則不能再被繼承者 override。
Out 參數修飾
讓引數變成 Reference Value,可以讓函數外的變數接收函數內的值;在使用 TryParse 時會用到。
int initializeInMethod;
OutArgExample(out initializeInMethod);
Console.WriteLine(initializeInMethod); // value is now 44
void OutArgExample(out int number)
{
number = 44;
}
string numberAsString = "1640";
int number;
if (Int32.TryParse(numberAsString, out number))
Console.WriteLine($"Converted '{numberAsString}' to {number}");
else
Console.WriteLine($"Unable to convert '{numberAsString}'");
// The example displays the following output:
// Converted '1640' to 1640
Readonly 修飾詞
可以保證 Field 只被初始化一次,並且不會重複初始化以免讓資料流失。
public class Customer
{
public readonly List<Order> Orders = new List<Order>(); //物件建構時,自動初始化
}
Null 條件運算子 (C#6)
C# Null Conditional Operator, Null 條件運算子
封裝 Field 與讀取 Property 的範例
public class Person
{
public DateTime BirthDate {get; set;}
public int Age {
get {
var timespan = DateTime.Now - BirthDate;
return timespan.Days / 365;
}
}
}
取得執行中物件的型別 (typeof / GetType)
p.GetType().Name
typeof (Point).Name
字串處理
集合類型
集合類型的初始建構 Collection initializers
List<int> digits = new List<int> { 0, 6, 1, 9 };
List<string> fruits = new List<string> { "Mango", "Lichee", "Lemon" };
List<Point> points = new List<Point> { new Point{X = 3, Y = 5}, new Point{X = 2, Y = 7} };
var dict = new Dictionary<int, StudentName>()
{
{ 017, new StudentName { Name = "sdwh", ID = 5017 } },
{ 028, new StudentName { Name = "phym", ID = 5028 } },
{ 099, new StudentName { Name = "mike", ID = 5099 } }
};
MSDN - Object and Collection Initializers (C# Programming Guide)
匿名類型建構 Anonymous Types
var pet = new { Age = 10, Name = "Fluffy" };
匿名類型的集合建構 List of Anonymous Types
var anonArray = new[] {
new { name = "apple", number = 4 },
new { name = "grape", number = 1 },
};
foreach (var fruit in anonArray)
{
Console.WriteLine($"{fruit.name} {fruit.number}");
}
索引子範例 Examples of Indexer
範例 1
public class HttpCookie
{
private readonly Dictionary<string, string> _dictionary;
public DateTime Expire {get; set;}
public HttpCookie()
{
_dictionary = new Dictionary<string, string>();
}
public string this[string key]
{
get { return _dictionary[key];}
set { _dictionary[key] = value;}
}
}
範例 2
class Sentence
{
string[] words = "The quick brown fox".Split();
public string this [int wordNum] // indexer
{
get { return words [wordNum]; }
set { words [wordNum] = value; }
}
}
static void Main()
{
Sentence s = new Sentence();
Console.WriteLine (s[3]); // fox
s[3] = "kangaroo";
Console.WriteLine (s[3]); // kangaroo
}
字典示範 (Examples of Dictionary)
var d = new Dictionary<string, int>();
d.Add("One", 1);
d["Two"] = 2; // adds to dictionary because "two" not already present
d["Two"] = 22; // updates dictionary because "two" is now present
d["Three"] = 3;
Console.WriteLine (d["Two"]); // Prints "22"
Console.WriteLine (d.ContainsKey ("One")); // true (fast operation)
Console.WriteLine (d.ContainsValue (3)); // true (slow operation)
int val = 0;
if (!d.TryGetValue ("onE", out val))
Console.WriteLine ("No val"); // "No val" (case sensitive)
// Three different ways to enumerate the dictionary:
foreach (KeyValuePair<string, int> kv in d) // One ; 1
Console.WriteLine (kv.Key + "; " + kv.Value); // Two ; 22
// Three ; 3
foreach (string s in d.Keys) Console.Write (s); // OneTwoThree
Console.WriteLine();
foreach (int i in d.Values) Console.Write (i); // 1223
Console.WriteLine();