ASP.NET Core CORS (Cross-Origin Resource Sharing)
2024-07-04
筆記 ASP.NET Core WebAPI 設定 CORS 的基本要點。
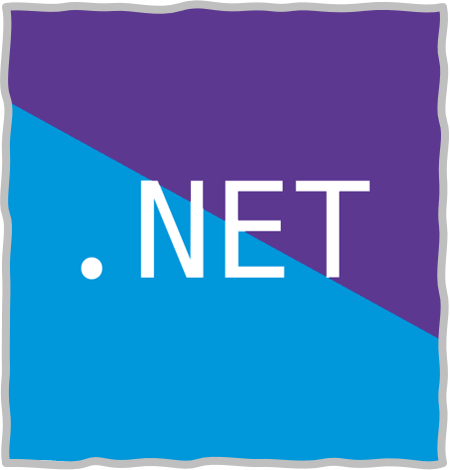
說明
What is CORS?
CORS (Cross-Origin Resource Sharing) is a security feature that allows web applications running on one domain to make requests to another domain. This is important because it allows web applications to access resources from other domains, which is necessary for building modern web applications.
Enable CORS in ASP.NET Core
Program.cs
builder.Services.AddCors(options =>
{
options.AddDefaultPolicy(builder =>
{
builder.AllowAnyOrigin()
.AllowAnyHeader()
.AllowAnyMethod();
});
});
var app = builder.Build();
app.UseCors(); // must before MapControllers
app.MapControllers();
app.Run();
To test CORS, we can using a simple HTML file to test.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Fetch Data from API</title>
<!-- Bootstrap 5 CSS -->
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<div class="container" id="container">
<h1 class="text-center my-3">Fetch Data from API</h1>
<div class="text-center my-3">
<button id="fetchDataBtn" class="btn btn-primary">Fetch Data</button>
</div>
</div>
<script>
document.getElementById('fetchDataBtn').addEventListener('click', function () {
fetch('https://localhost:7055/api/Todo')
.then(response => response.json())
.then(data => {
// Check if the table already exists, if so, remove it
let existingTable = document.querySelector('.table');
if (existingTable) {
existingTable.remove();
}
// Create a new table
const table = document.createElement('table');
table.className = 'table table-hover';
table.innerHTML = `
<thead class="table-dark">
<tr>
<th>ID</th>
<th>Name</th>
<th>Is Complete</th>
</tr>
</thead>
<tbody>
</tbody>
`;
const tbody = table.querySelector('tbody');
data.forEach(item => {
const row = document.createElement('tr');
row.innerHTML = `
<td>${item.id}</td>
<td>${item.name}</td>
<td>${item.isComplete ? 'Yes' : 'No'}</td>
`;
tbody.appendChild(row);
});
const container = document.getElementById('container');
container.appendChild(table);
})
.catch(error => console.error('Error:', error));
});
</script>
</body>
</html>