.NET 使用初體驗,本次使用 .NET Core 3.1 並且使用 Razor Pages 專案,實現以往在 .NET Framework 的 system.web.helpers serverinfo
功能。
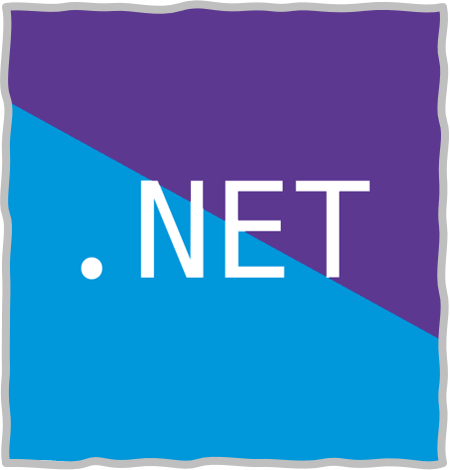
說明
意外的發現 .NET Core 沒有與 .NET Framework 相似功能的 serverinfo,我難過,真心覺得這個功能好用。
正好前一陣子學習 AZ-204 時,接觸了 .NET Core 但卻沒有留下太深刻的記憶,想必是缺乏實作的關係,本次藉此機會實作 .NET Core 使用 Razor Pages 專案,實作的目標則是相似於原本 .NET Framework 所提供的 serverinfo
功能。
Coding
在 .NET Core 的精巧架構下,尤其是 Razor Pages 當中,只使用必要的功能以保持絕佳的效能。在預設上 Session 並沒有啟用,開發者必須主動使用。
Startup.cs
使用的方式是在 Startup.cs 下註冊服務以及使用,Session 的逾期時間比照 .NET Framework 的預設為 20分鐘 (1200秒) 🙂
public void ConfigureServices(IServiceCollection services)
{
services.AddRazorPages();
services.AddSession(options => {
options.IdleTimeout = System.TimeSpan.FromSeconds(1200);
});
services.AddSingleton<IHttpContextAccessor, HttpContextAccessor>();
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseSession();
}
index.cshtml.cs
OnGet 主要處理伺服器端的 Env 變數,整理為 Dictionary 提供 View 使用,所以需要宣告屬性 EnvProperties
提供 View 以 Model 的方式使用。
另外測試 Cookie 以及 Session 的寫入,其中在 Session 的寫入上,必須要將 String 轉為 Bytes,這邊使用的是 Encoding.ASCII.GetBytes
public class IndexModel : PageModel
{
private readonly ILogger<IndexModel> _logger;
public IndexModel(ILogger<IndexModel> logger)
{
_logger = logger;
}
public Dictionary<string, string> EnvProperties { get; set; }
public void OnGet()
{
Response.Cookies.Append("Wafer", "Small");
Response.Cookies.Append("Oreo", "Medium");
HttpContext.Session.Set("Identity", Encoding.ASCII.GetBytes("WinAuth"));
var envType = typeof(Environment);
var properties = envType.GetProperties();
EnvProperties = new Dictionary<string, string> { };
foreach (var env in properties)
{
EnvProperties.Add(env.Name, env.GetValue(env.Name).ToString());
}
}
}
index.cshtml
如果在 View 要讀取 Session 的資料,需要搭配 using 以及 inject 引入相關的 library。
@page
@model IndexModel
@using Microsoft.AspNetCore.Http;
@inject Microsoft.AspNetCore.Http.IHttpContextAccessor HttpContextAccessor;
@{
ViewData["Title"] = "Home page";
}
<div class="">
<div class="card px-3 py-1">
<h2>Response</h2>
<dl>
@foreach (var header in Response.Headers)
{
<dt>@header.Key</dt>
<dd>@header.Value</dd>
}
</dl>
</div>
<div class="card px-3 py-1 mt-3">
<h2>Requests</h2>
<dl>
@foreach (var header in Request.Headers)
{
<dt>@header.Key</dt>
<dd>@header.Value</dd>
}
</dl>
</div>
<div class="card px-3 py-1 mt-3">
<h2>Cookies</h2>
<dl>
@foreach (var header in Request.Cookies)
{
<dt>@header.Key</dt>
<dd>@header.Value</dd>
}
</dl>
</div>
<div class="card px-3 py-1 mt-3">
<h2>Sessions</h2>
<div>@HttpContext.Session.Id</div>
<dl>
@foreach (var key in HttpContext.Session.Keys)
{
<dt>@key</dt>
<dd>@HttpContext.Session.GetString(key)</dd>
}
</dl>
</div>
<div class="card px-3 py-1 mt-3">
<h2>Environment</h2>
<dl>
@foreach (var env in Model.EnvProperties)
{
<dt>@env.Key</dt>
<dd>@env.Value</dd>
}
</dl>
</div>
</div>