使用 ASP.NET MVC 開發時,最常見的痛點就是在設計表單 (forms) 需要來回切換頁面查詢 Bootstrap 的架構格式,雖然有 Snippets 可以使用,但仍是有許多地方需要進行客製,同時也要考慮到 ASP.NET MVC 提供了許多的 Helper,不善加利用十分可惜,於是綜合兩者的優勢的開發方式以及常用的 Code Helper 及 Snippets 整理於本筆記,供日後檢索。
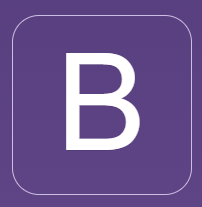
常用元件
表單架構
<div class="container">
<h2>Heading</h2>
@using (Html.BeginForm())
{
@Html.AntiForgeryToken()
<div class="form-horizontal">
<hr />
@Html.ValidationSummary(true, "", new { @class = "text-danger" })
@Html.HiddenFor(model => model.Id)
<div class="form-group">
...
</div>
<div class="form-group">
<input type="submit" value="搜尋" class="btn btn-primary mt-3">
</div>
</div>
}
</div>
未使用 Helper 的方式
<form action="~/Controller/Action" method="post">
<div class="form-horizontal">
...
</div>
</form>
Text Input (文字輸入)
<div class="form-group">
@Html.LabelFor(model => model.Property, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Property, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Property, "", new { @class = "text-danger" })
</div>
</div>
Number Input (數值輸入)
View 的程式碼與 Text Input 相同,Html Helper 會根據 Model 中的型別自動渲染成不同的 Input Attribute。
Date Input (日期輸入)
Textarea Input (長文輸入)
Navbar Search (搜尋輸入)
Radio Button (單選按鈕)
<div class="form-group">
@Html.LabelFor(model => model.Active, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
<label class="radio-inline">
<input type="radio" name="Active" id="radio1" value="true" checked="@(Model.Active == "true")"> 啟用
</label>
<label class="radio-inline">
<input type="radio" name="Active" id="radio1" value="false" checked="@(Model.Active != "true")"> 停用
</label>
@Html.ValidationMessageFor(model => model.Active, "", new { @class = "text-danger" })
</div>
</div>
Checkbox (複選按鈕)
<div class="form-group">
@Html.LabelFor(model => model.Property, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
<label class="checkbox-inline">
<input type="checkbox" name="Active" id="ActiveRadio1" value="true")"> 啟用
</label>
@Html.ValidationMessageFor(model => model.Property, "", new { @class = "text-danger" })
</div>
</div>
Selection List (下拉選單)
取代範例程式碼中的 Table
及 Property
。
/Controller/Controller.cs
[NonAction]
public void ListSlectionGenerator()
{
List<SelectListItem> mySelectItemList = new List<SelectListItem>()
{
new SelectListItem() { Text = "請選擇類別或於右側欄位輸入自訂項目", Selected = true}
};
foreach (var item in db.Table.GroupBy(i => i.Property))
{
mySelectItemList.Add(new SelectListItem()
{
Text = item.Key,
Value = item.Key,
});
}
ViewBag.DropDownListForProperty = mySelectItemList;
}
/View/cshtml
@model NameSpace.Models.ClassName
<div class="form-group">
@Html.LabelFor(model => model.Property, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
<div class="col-md-5 pl-0">
@Html.DropDownList(
"PropertyDropdown",
(IEnumerable<SelectListItem>)ViewBag.DropDownListForProperty,
new { @class = "form-control" })
</div>
<div class="col-md-5">
@Html.EditorFor(
model => model.Property,
new {
htmlAttributes = new {
@class = "form-control",
placeholder = "自訂並新增類別",
}
})
</div>
@Html.ValidationMessageFor(model => model.Property, "", new { @class = "text-danger" })
</div>
</div>
@section scripts{
<script>
$('#ComponentDropdown').change(function () {
$('#Component').val($('#ComponentDropdown').val());
});
</script>
}